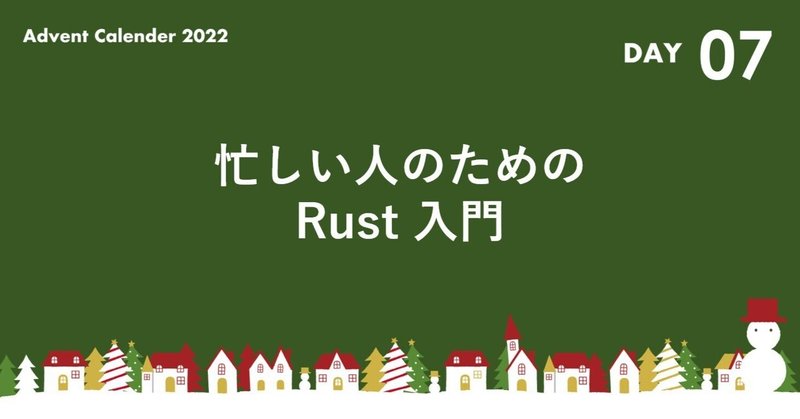
忙しい人のためのRust入門
はじめに
こんにちは!DAAE部 開発グループ所属の白木です。
年末で忙しいけど「最近よく Rust を聞くからサクッとふれてみたい」という方向けに Rust 入門の記事を書きました。
Rust での開発の流れを体験してもらうことを想定しており、言語仕様などの説明はありません。
ドキュメントへのリンクや参照元を各所に記載しているので、気になったらそこから掘りさげてもらえると嬉しいです。
なぜ Rust に入門するのか?
JavaScript/TypeScript まわりで Rust で作られたツールを目にする機会が増えてきました。
他にも
Linux (v6.1 で一部に入る予定)
ターミナルアプリの Warp
など Rust を使って開発されたソフトウェアが出てきています。
これらを使う分には Rust を意識することはないのですが、問題が起きたときの調査やカスタマイズしたいときは Rust になじみがあれば解決がはやまります。
「近い将来ぶつかるであろう課題にそなえて Rust にふれておく」が入門の理由です。
実行環境
この記事では以下の環境で動作を確認しています。
$ uname -ms
Darwin arm64
$ sw_vers
ProductName: macOS
ProductVersion: 12.6.1
BuildVersion: 21G217
環境構築
Rust の開発環境を構築するために rustc と Cargo をインストールします。
rustc とは?
Rust のコンパイラです。
参考: What is rustc?
Cargo とは?
Rust のパッケージマネージャー (Node.js でいう npm) です。
参考: The Cargo Book
Rust の開発では rustc を使うことは少なく Cargo を通じて rustc を呼び出します。
Cargo 内部で rustc をどのように使っているか知りたいときは --verbose オプションをつけて実行すると出力されます。
インストール
# インストール
$ curl --proto '=https' --tlsv1.2 -sSf https://sh.rustup.rs | sh
# PATHの再読み込み
$ source "$HOME/.cargo/env"
$ rustc --version
rustc 1.65.0 (897e37553 2022-11-02)
$ cargo --version
cargo 1.65.0 (4bc8f24d3 2022-10-20)
rustc と Cargo が使えるようになりました!
VS Code を使っている場合は rust-analyzer も入れておきましょう。
Rust プロジェクトの作成
cargo new を使って Hello world! を出力する Rust プロジェクトを作成します。
# プロジェクトの作成
$ cargo new rust-hello-world
$ cd rust-hello-world
# 生成されたファイルの確認
$ tree
.
├── Cargo.toml
└── src
└── main.rs
Hello world! を出力する main.rs が生成されました。
Rust の実行時には main 関数が最初に実行されます。
// src/main.rs
fn main() {
println!("Hello, world!");
}
cargo run で実行します。
# コンパイルと実行
$ cargo run
Hello, world!
Rust が動きました!
--verbose オプションを付けると、rustc で main.rs をコンパイルした後に生成されたバイナリファイル(target/debug/rust-hello-world)を実行していることがわかります。
$ cargo run --verbose
Compiling rust-hello-world v0.1.0 (/path/rust/rust-hello-world)
Running `rustc --crate-name rust_hello_world --edition=2021 src/main.rs --error-format=json --json=diagnostic-rendered-ansi,artifacts,future-incompat --crate-type bin --emit=dep-info,link -C embed-bitcode=no -C split-debuginfo=unpacked -C debuginfo=2 -C metadata=e07b0cec3edba003 -C extra-filename=-e07b0cec3edba003 --out-dir /path/rust/rust-hello-world/target/debug/deps -C incremental=/path/rust/rust-hello-world/target/debug/incremental -L dependency=/path/rust/rust-hello-world/target/debug/deps`
Finished dev [unoptimized + debuginfo] target(s) in 0.37s
Running `target/debug/rust-hello-world`
Hello, world!
Rust でバックエンド開発
つづいて Web フレームワークを使ってシンプルなバックエンドの API をつくります。
rust-web-framework-comparison をみると Actix Web, axum, Rocket あたりが良さそうです。
今回は、開発が活発で安定バージョン(v1.0)に到達している Actix Web を使います。
# プロジェクトの作成
$ cargo new rust-backend
$ cd rust-backend
# Actix Web をプロジェクトに追加
$ cargo add actix-web
main.rs を以下のコードに書き換えます。
// src/main.rs
use actix_web::{get, post, App, HttpResponse, HttpServer, Responder};
#[get("/")]
async fn hello() -> impl Responder {
HttpResponse::Ok().body("Hello world!")
}
#[post("/echo")]
async fn echo(req_body: String) -> impl Responder {
HttpResponse::Ok().body(req_body)
}
#[actix_web::main]
async fn main() -> std::io::Result<()> {
HttpServer::new(|| {
App::new()
.service(hello)
.service(echo)
})
.bind(("127.0.0.1", 8080))?
.run()
.await
}
#[xxx] はアトリビュートと呼ばれ、関数に対してメタデータ(機能)を追加します。
#[get("/")], #[post("/echo")]では HTTP メソッドとパスのルーティングを、#[actix_web::main] ではサーバーの初期化・実行の機能を関数に追加しています。
cargo run でサーバーを起動して、API の動作確認をします。
# サーバーを起動
$ cargo run
Compiling rust-backend v0.1.0 (/path/rust/rust-backend)
Finished dev [unoptimized + debuginfo] target(s) in 27.26s
Running `target/debug/rust-backend`
# cargo run とは別のターミナルで実行
$ curl http://127.0.0.1:8080/
Hello world!
# cargo run とは別のターミナルで実行
$ curl http://127.0.0.1:8080/echo -X POST -d '{"foo":"bar"}'
{"foo":"bar"}
Rust でバックエンド API が動きました!
まとめ
Rust の環境構築、Hello world、バックエンド実装までを紹介しました!
他にも Rust の用途で一番多いライブラリ開発やフロントエンド開発も Rust 入門としての紹介を考えています。
さて、明日の「SHIFTアドベンドカレンダー」は
どんな記事が公開されるでしょうか?お楽しみに!
\もっと身近にもっとリアルに!DAAE公式Twitter/
執筆者プロフィール: Shoya Shiraki
ソフトウェアエンジニアとしてソーシャルゲーム開発、スタートアップでの CTO 経験を経て SHIFT に入社。 テクノロジーを人に最適化するをモットーに、日々楽しんで開発しています。
お問合せはお気軽に
https://service.shiftinc.jp/contact/
SHIFTについて(コーポレートサイト)
https://www.shiftinc.jp/
SHIFTのサービスについて(サービスサイト)
https://service.shiftinc.jp/
SHIFTの導入事例
https://service.shiftinc.jp/case/
お役立ち資料はこちら
https://service.shiftinc.jp/resources/
SHIFTの採用情報はこちら
https://recruit.shiftinc.jp/career/